Google Play is a platform that allows developers to publish their apps and games on the Android operating system. The process of publishing an app on the store is a long one, with many steps involved. This article will show you how to prepare your Flutter application for publication on Google Play Store.
The google play console is a tool that allows developers to publish their apps on the Google Play Store. In order to prepare for publishing, make sure your app is ready and follow these steps.
We must provide our software a digital signature by signing it before we can publish it on Google Play Store. Also, instead of the default flutter icon, we need to supply a nice launcher icon for the app and double-check that the package name, usage permission, and other variables are set properly.
So, if you’ve arrived here, I’m assuming you’ve finished your app and it’s functioning properly. Now you must do some more work in order to publish your app on the Google Play Store. Before releasing your app on the Play Store, there are two main procedures to take: adding a launcher icon and creating a signed App Bundle.
From August 2023, Google will make it obligatory to publish on the Play Store using App Bundles. This implies that the user must consent to Google Play App Signing.
Incorporating a Launcher Icon
You’ve created a flutter app and noticed a default launcher icon with the flutter logo. The icon that appears in the Android launcher is known as the launcher icon.
Adding a launcher icon may be a time-consuming procedure, but there is a program called flutter launcher icons that automates the process by performing all of the work for you. All of the instructions you’ll need to create a launcher icon are included in that bundle. I’ll also go over some key points here.
To begin, create a launcher icon in your flutter app. It’s the same process as adding assets or pictures. Assume icon.png is located in assets/icon/.
Then, in your pubspec.yaml file, put your Flutter Launcher Icons settings. Remember to use the most recent version.
flutter launcher icons: “0.8.0” in dev dependencies true image path: “assets/icon/icon.png” flutter icons: android: “launcher icon” ios: true image path: “assets/icon/icon.png”
YAML is the programming language (yaml)
Run the package once you’ve finished configuring it.
flutter pub flutter launcher icons:main flutter pub flutter pub flutter pub flutter pub flutter pub flutter pub flutter pub flutter pub f
YAML is the programming language (yaml)
After that, all of the files for your new launcher icon are created and setup. When you create and install the program again, you’ll see that the default launcher icon has been changed with a new one.
Adding a Signature to Your Flutter App
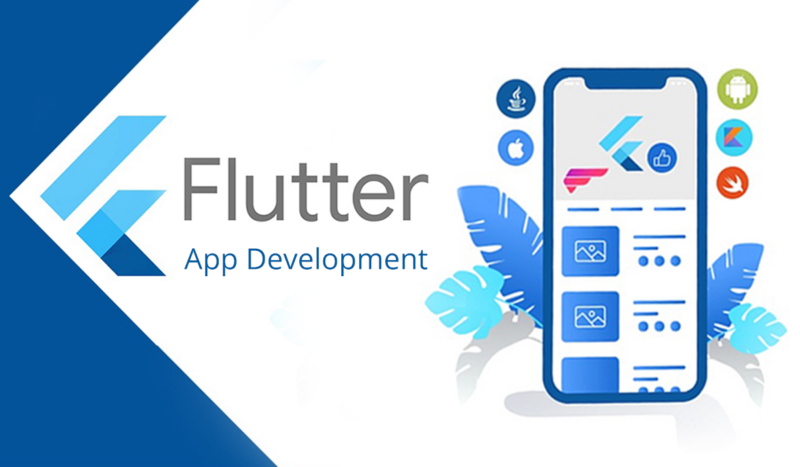
We can’t publish App Bundle on Google Play Store without signing it, and we can’t even install an unsigned APK on a device without signing it. But how is it feasible when you’ve already installed your flutter app while developing? Your program is already signed with a debug key, therefore the answer is yes. The debug key is included with the Android SDK, and while debugging, the APK is signed using the debug key by default. By the way, the debug keystore password is android; you don’t need it right now, but I informed you just in case. Perhaps you will need it in the future.
Take a look at the /android/app/build.gradle file. Then you’ll see something similar to this:
signingConfig signingConfigs.debug buildTypes release
Gradle is a programming language (gradle)
However, in order to publish an app on the Play Store, it must be signed with a private key that represents you and kept secret. You may check at the answers on Stackoverflow – Why should I sign my apk before publishing it to the PlayStore?
One essential aspect of app signing is that future upgrades of this app must be signed with the same prior private key. You won’t be able to publish a new update to that app if you lose the keystore or forget the password. However, in certain situations, you can recover your key if you lose your keystore (I haven’t tried it myself and don’t want to) – I lost my.keystore file?
Because we can’t update our program without the key we used to sign it, if you’re creating applications for others, you should always use a separate key. Using a separate key is always preferable since you won’t have to reveal your private key and password if I need to quit your business or sell that program to a different developer in the future.
So, if you’re working for others or need/plan to sell the app in the future, I recommend using a separate key, and you should really consider having one key per app. Personally, I always build a new keystore for each new project, save it outside the project location in a secure area, and then transfer the required information within the project. This is how the folder structure appears.
upload keys com.first.app upload-keystore.jks key.properties com.second.app key.properties upload-keystore.jks com.third.app key.properties upload-keystore.jks com.fourth.app key.properties upload-keystore.jks
Shell Session is a programming language (shell)
This manner, I won’t forget or lose my password or keystore, and I’ll be able to simply transfer/sell my published applications to another developer. Also, be careful not to share this with the public, or update the.gitignore file to exclude the folder or files from version control.
So I’ve covered all you need to know before signing an App Bundle/APK. Now we’ll use keytool to create a private key and keystore, and then we’ll sing our app.
Make a keystore for uploading
Make a folder to keep all of your keys in. Then create a new folder with the package’s name in it. This manner, we can quickly identify which key belongs to which program.
Go to the location where you want to build the keystore and run the command:
Shell Session is a programming language (shell)
The key.jks file is saved in your current working directory using this command. Change the argument you give to the -keystore option if you wish to store it somewhere else. Remember the alias, upload; we’ll use it later in the key.properties file.
When establishing a keystore, you’ll be asked to provide some personal information as well as a password for both the keystore and the key. We may use the same key password as keystore for convenience. If you wish to use the same key password as the keystore password, you’ll be prompted. Below is a snapshot taken when building a keystore for one of my demo apps.
Since it is just for learning purposes, I have just typed my app name everywhere. However, you must provide accurate information about yourself, such as your actual name, nationality, and so on. Also, store this key in a secure location, don’t post it somewhere public, and don’t forget the password. Your private key is secure until the password is unknown to others, even if you posted it to public sites or if your key is stolen. You don’t want to take a chance since they can bruteforce or guess. Is the RSA private key worthless if the password isn’t entered?
Create a key.properties file with a keystore reference
Create a file called key.properties in the same directory as upload-keystore.jks and fill it with keystore information like this:
keyAlias=upload storePassword= keyPassword= storeFile=../upload-keystore.jks
HTML and XML are the two types of code that may be used (xml)
If my password is myPassword123, it will appear as follows:
store key=myPassword123 password=myPassword123 password=myPassword123 password=myPassword keyAlias=upload store password=myPassword123 File=../upload-keystore.jks
This file must be read from the app level build.gradle, which is my flutter project/android/app/build.gradle. We subsequently put both key.properties and upload-keystore.jks into the android folder, which is why storeFile is../upload-keystore.jks.
Complete the app’s referring to the keystore file.
Inside /android/, copy both key.properties and upload-keystore.jks.
Keep the key secure. Make the properties file private, and don’t check it into source control. To do so, place this file in the android directory’s.gitignore file. Those files will then be grayed out.
key.properties upload-keystore.jks
CSS is the programming language (css)
Gradle may be used to configure signing
Edit the /android/app/build.gradle file to configure signing for your app.
Add the following before the android block to import the key.properties file into the keystoreProperties object:
JavaScript is the programming language used in this project (javascript)
Then it appears as follows:
Also, before the buildTypes block, add the signing configurations information, and change signingConfig signingConfigs.debug to signingConfig signingConfigs.release within the buildTypes block.
Gradle is a programming language (gradle)
Then it should seem as follows:
When you generate an App Bundle or APK, it will now be automatically signed with the release key.
Examining the manifest file
/android/app/src/main/AndroidManifest.xml is the location of the App Manifest file. Before publishing an app, ensure sure it has the proper name and package name specified at android:label in the application tag. com.example is the default package name. As a result, you don’t want that package name to create any issues in the future. I don’t go into detail on how to alter the package name here. This answer can be found on Stackoverflow: How to modify the package name in flutter.
Your app may also need internet access or other permissions. It is not required to add internet permission to the Application Manifest in debug mode for your program to access the internet. However, in order to operate correctly in release mode, the app must have the uses permission added. Add the outside application tag to your app to provide internet authorization. Other permissions may be added in the same manner.
Examining the construction parameters
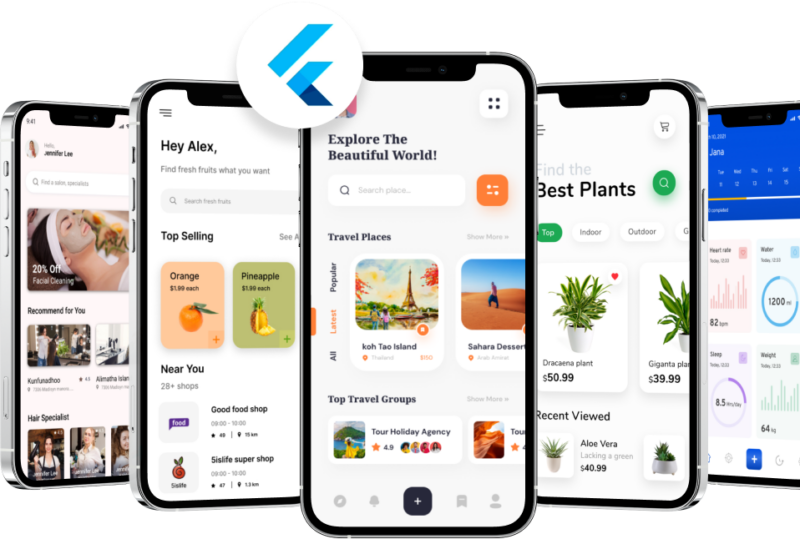
Examine the default Gradle build file, build.gradle, found in /android/app, and double-check that the settings are accurate, particularly the defaultConfig block values:
- The package name as specified in the AndroidManifest.xml file is applicationId.
- verisonName and versionCode
- targetSdkVersion, minSdkVersion, and compileSdkVersion
You may now create your APK or App Bundle. Only App Bundles will be able to be submitted to the Play Store Console after August 2023. If you want to learn more about App bundle, you may watch a video about it.
Run flutter build appbundle to create an app bundle, and flutter build apk —split-per-abi to create an APK.
Visit – Build and release an Android app for additional information on APK splitting, building techniques, and official documentation related to this topic.
When publishing an Android app in the Play Store interface, Google does not accept APKs; instead, appbundles are needed.
App Integrity After Publication
We’ve finally uploaded the app to the Google Play Store. Google will handle your app signing key if you have chosen to use Play App Signing. It implies that Google, not you, will sign the app for the user. So, where did our key go that we signed before uploading? The key used to sing the APK is solely needed to submit the APK to Play Console, allowing Play Console to recognize your identity.
Assuming you’re using a service like Firebase or Facebook for authentication, they’ll need a sha-1 key or a key hash of the app signing key. Because Google signed the final APK, these services will no longer function in your app. The sha-1 key may be found on the App Integrity page of the Play Store Console.
The Facebook Developer Console needs a base 64 key hash, which may be created using sha-1.
echo xxd -r -p | openssl base64 | YOUR SHA-1 (Hexadecimal)
Bash is the programming language (bash)
The original Stack Overflow response is as follows: https://stackoverflow.com/a/52785464/8880002
I hope you found this post to be informative.
The how to launch an app on google play store is a guide that will help you prepare your Flutter App for publishing on the Google Play Store.
Frequently Asked Questions
How do you deploy a flutter app on Android?
Flutter is a cross-platform mobile app development framework. The following command will deploy your app to the emulator on your computer.
How do you make your own app and put it on the Play Store?
To make your own app, you need to create a new project in Android Studio.
How do I release the app flutter app?
To release the app, you have to press and hold the power button for 10 seconds.